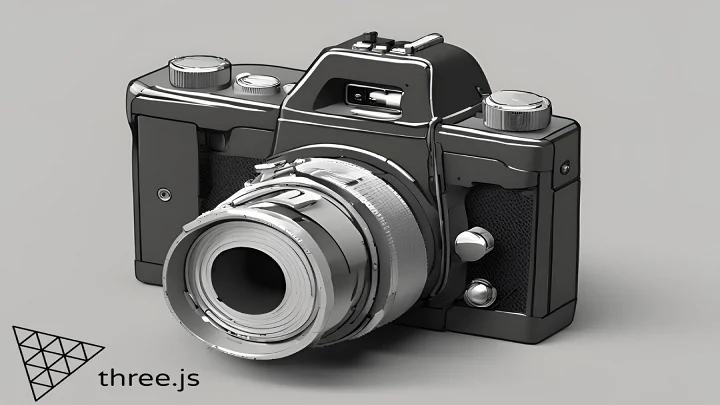
Three.js Orthographic Camera Basics, Setting Up and Configuring
The orthographic camera is a fundamental component in Three.js, allowing developers to create stunning 3D visualizations. In this post, we will delve into the basics of setting up and configuring the orthographic camera in Three.js, covering its properties, and providing practical examples to get you started.
Want to take your Three.js skills to the next level? Explore our comprehensive roadmap of Three.js courses and start building stunning 3D visualizations today! Three.js roadmap
Setting Up the Orthographic Camera
When setting up the orthographic camera, you typically don't add it directly to the scene like other objects. Instead, you set it as the active camera for rendering. Also, ensure you import THREE
properly if you're using ES6 modules.
import * as THREE from 'three';
// Assuming 'scene' is already defined
const aspect = window.innerWidth / window.innerHeight;
const d = 10;
const camera = new THREE.OrthographicCamera(
-d * aspect,
d * aspect,
d,
-d,
0.1,
1000
);
// Set the camera as the active one for rendering
renderer.camera = camera;
Configuring Camera Properties
The orthographic camera has several properties that can be configured to suit your specific needs. Here are some of the most important ones:
- left: The left coordinate of the camera's frustum.
- right: The right coordinate of the camera's frustum.
- top: The top coordinate of the camera's frustum.
- bottom: The bottom coordinate of the camera's frustum.
- near: The near clipping plane distance.
- far: The far clipping plane distance.
Here's an example of how to configure these properties:
camera.left = -10;
camera.right = 10;
camera.top = 10;
camera.bottom = -10;
camera.near = 0.1;
camera.far = 1000;
camera.updateProjectionMatrix(); // Apply changes
Camera Positioning
Positioning the camera is straightforward, but ensure you're modifying the camera's position relative to the scene's coordinate system.
camera.position.set(0, 0, 5);
Camera Rotation
Rotating the camera involves Euler angles (in radians), so ensure you're using the correct units. Also, note that rotations in Three.js are applied around the local axes, not the world axes.
camera.rotation.set(0, Math.PI / 4, 0);
Camera Up Vector
Setting the camera's up vector is useful for defining the "up" direction, especially when looking at targets that aren't aligned with the world's Y-axis.
camera.up.set(0, 1, 0); // Typically, the Y-axis is considered "up"
Camera Look At
Using lookAt
to orient the camera towards a specific point is common. Ensure you're passing a THREE.Vector3
object as the target.
camera.lookAt(new THREE.Vector3(0, 0, 0));
In this post, we covered the basics of setting up and configuring the orthographic camera in Three.js. By understanding its properties and fundamental concepts, you can effectively implement the orthographic camera in your projects. For more advanced techniques and best practices, check out our roadmap of Three.js courses at Three.js course guide.